|
CS and Mathematics @ BYU
I write about code, AI/ML, and whatever catches my eye.
Featured Work
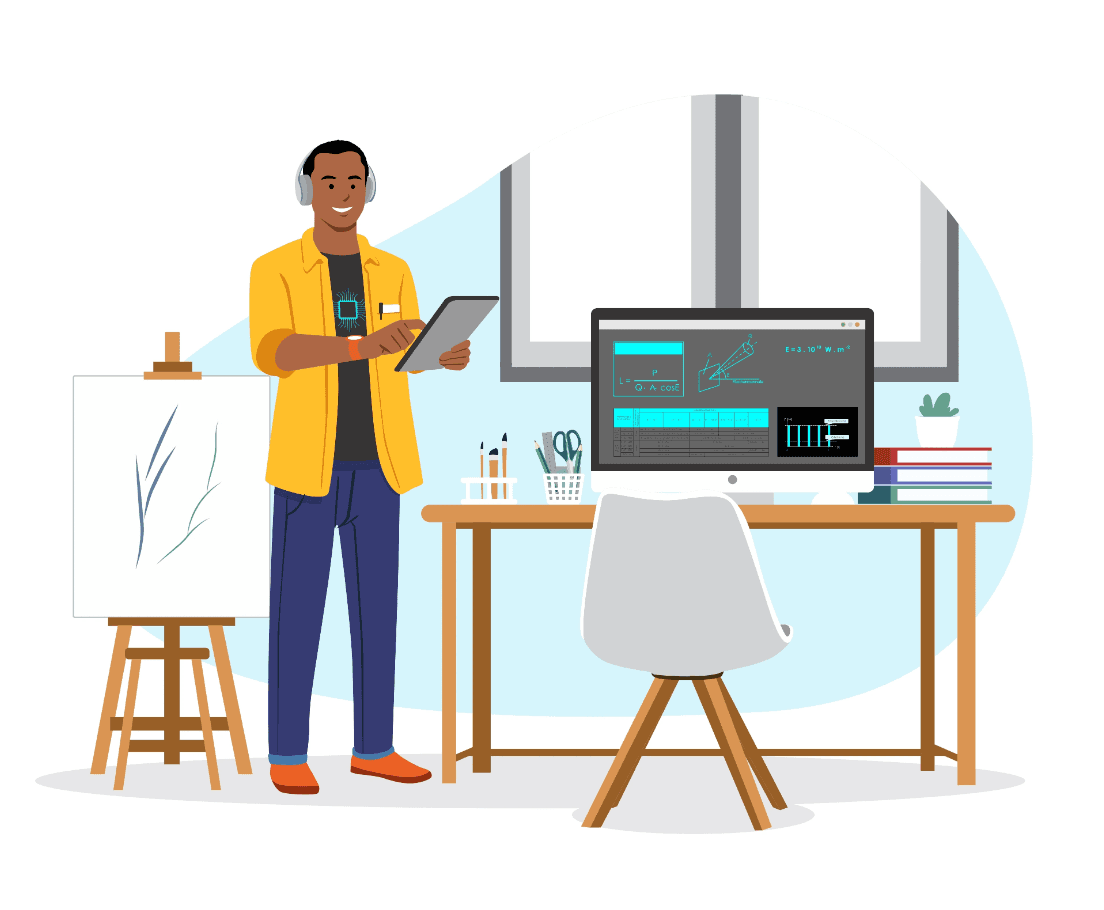
project
Style Canvas
December 30, 20244 min read
A full-stack platform for hosting AI models with on-demand GPU inference, JWT auth, and a simple token system. Built to be fast, scalable, and cheap.
Model DeploymentWeb DevAI/ML
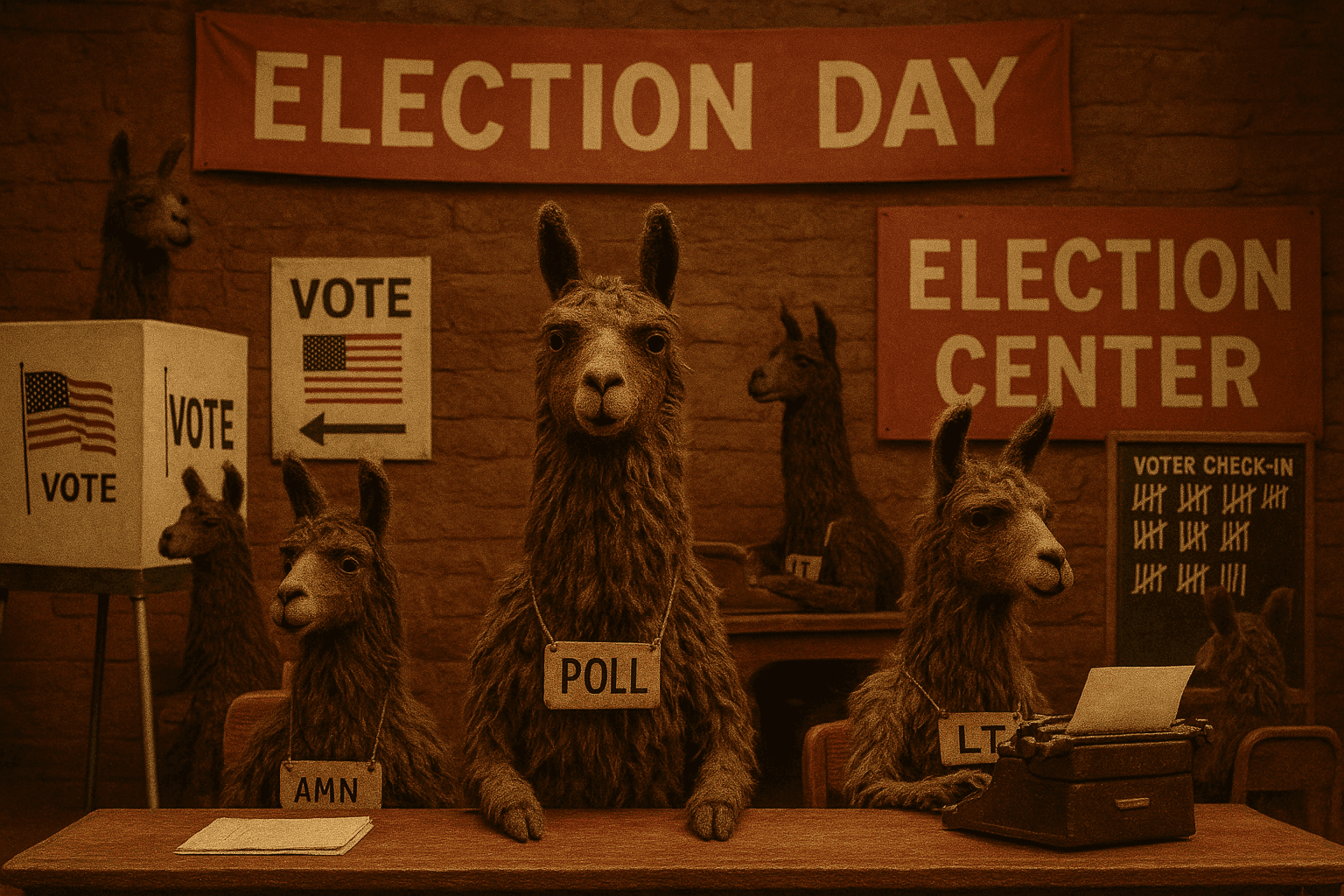
research
LLM Generated Distribution-Based Prediction of US Electoral Results, Part I
November 4, 20243 min read
This paper introduces Distribution-Based Prediction, a method for interpreting LLM output probabilities as predictive distributions. Applied to US elections, it enables analysis of model bias, prompt noise, and algorithmic fidelity.
LLMsElectionsCalibrationBiasEvaluationMachine Learning
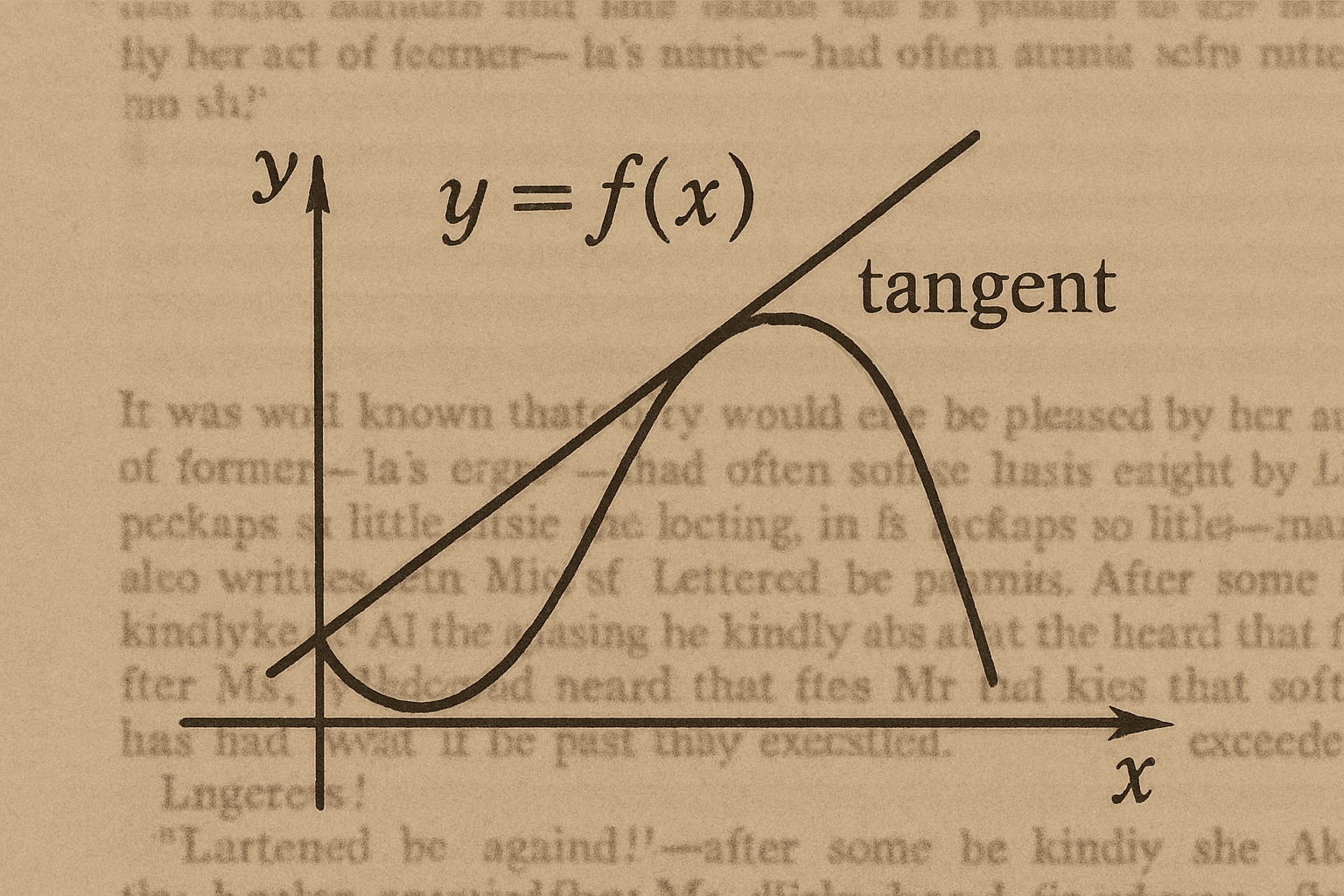
research
Taking the Derivative of a Story: A Novel Approach to Fiction Scene Segmentation
November 1, 20242 min read
This paper introduces a new method for scene segmentation in fiction by calculating a 'derivative' over sentence embeddings, using local minima as candidates for scene transitions.
Scene SegmentationNarrative AnalysisEmbeddingsNLP